Exploring Multithreading in Python: A Simple Guide with Examples
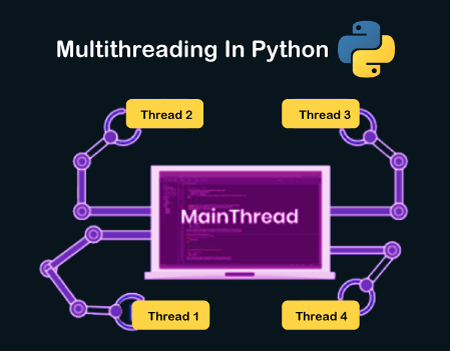
Multithreading is a powerful concept in computer science that allows programs to execute multiple threads (smaller units of a process) concurrently within a single process. This means that a program can perform multiple tasks at the same time, improving efficiency and responsiveness. In this article, we will take a look into the world of multithreading using Python.
Understanding Multithreading
In traditional programming, tasks are executed sequentially. If a task takes a significant amount of time to complete, it might lead to delays and reduced performance. Multithreading addresses this by breaking down a program into smaller threads, each handling a specific task. These threads can run concurrently on different CPU cores, enabling faster execution and better resource utilization.
Python’s threading
module provides a simple way to work with threads. Keep in mind that Python's Global Interpreter Lock (GIL) restricts the execution of multiple threads in a single process, so multithreading might not fully utilize multiple cores in CPU-bound tasks. However, it's still effective for I/O-bound tasks where threads spend time waiting for external resources.
A Multithreading Example in Python
Let’s consider a scenario where we need to download images from multiple URLs. This is an ideal use case for multithreading, as downloading involves waiting for external resources (I/O-bound).
Installation
Before we begin, ensure you have Python installed on your system.
Example Code
import threading
import requests
from PIL import Image
from io import BytesIO
# List of image URLs to download
image_urls = [
"https://example.com/image1.jpg",
"https://example.com/image2.jpg",
"https://example.com/image3.jpg",
# Add more URLs here
]
# Function to download an image from a URL
def download_image(url):
response = requests.get(url)
image = Image.open(BytesIO(response.content))
image.save(f"{url.split('/')[-1]}.jpg")
print(f"Downloaded: {url}")
# Create thread objects
threads = []
for url in image_urls:
thread = threading.Thread(target=download_image, args=(url,))
threads.append(thread)
thread.start()
# Wait for all threads to finish
for thread in threads:
thread.join()
print("All downloads completed.")
Explanation
- We import the necessary modules:
threading
for working with threads,requests
for making HTTP requests,PIL
(Pillow) for handling images, andBytesIO
for working with binary data. - We define a list of image URLs that we want to download.
- The
download_image
function takes a URL as an argument, and downloads the image usingrequests.get()
, and saves it to disk using theImage
class from PIL. - We create thread objects for each image URL. The
target
parameter specifies the function to be executed in the thread, and theargs
parameter provides the function's arguments. - We start each thread using the
start()
method. - We wait for all threads to finish using the
join()
method. This ensures that the main program waits for all threads to complete before proceeding. - After all threads are finished, we print a completion message.
Running the Example
- Save the example code in a
.py
file. - Install the required packages using
pip install requests Pillow
. - Run the script using
python script_name.py
.
Conclusion
Multithreading in Python can significantly improve the performance of I/O-bound tasks by allowing concurrent execution of threads within a single process. While the Global Interpreter Lock restricts the full utilization of multiple CPU cores for CPU-bound tasks, multithreading remains a valuable technique for responsive and efficient I/O-bound applications. As you explore more complex scenarios, remember to manage thread synchronization and be mindful of potential race conditions to ensure the stability of your multithreaded programs.
That’s it for this article! Feel free to leave feedback or questions in the comments.
Enjoyed the article and found it helpful? If you’d like to show your appreciation and support my work, you can buy me a coffee! Your support goes a long way in helping me create more content like this. Thank you in advance! ☕️