Understanding Test Driven Development (TDD) and Its Workflow
1. What is TDD (Test Driven Development)?
Before we discuss what TDD is, let’s think about the traditional coding approach.
Usually, we think about the function we want to create first, then write the code, and finally test it to see if it works.
However, the TDD workflow is the opposite. We are writing automated tests before writing the actual code. Instead of writing the code first and then trying to create a test to verify it.
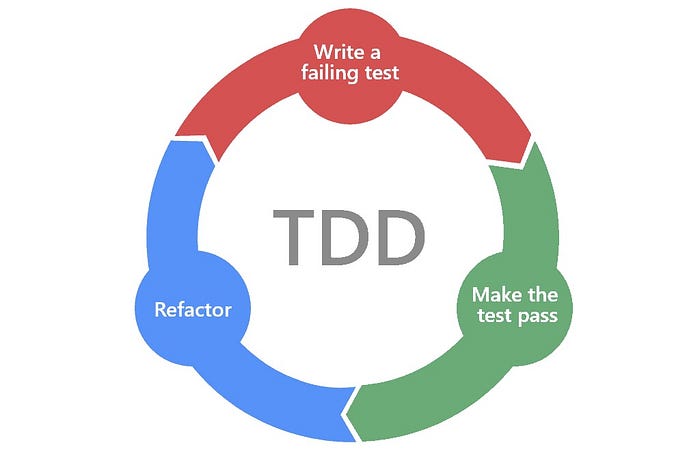
- Write a test
2. Write the actual code
3. Test it to pass
4. Refactorize if necessary
Before we dive into TDD, I want to tell you about the various types of automated tests that can be written:
- Unit tests: These tests focus on testing individual functions that perform specific tasks. Unit testing is relatively simple to get started with. You call a single function and check the result to ensure its correctness.
- Integration tests: These tests verify that unit collaborations work correctly. In integration testing, you typically check the interaction and integration between multiple units of code simultaneously. It ensures that different components or modules work together as expected.
- End-to-end tests: These tests examine the entire application or system as a whole, simulating real user interactions. End-to-end testing aims to validate the flow and functionality of the application from start to finish. It ensures that all the components and systems collaborate seamlessly, mimicking real-world scenarios.
2. What are the Benefits of TDD?
TDD offers not only assistance in developing the logic in our code but also a couple of other benefits.
- Focused Development
TDD enables you to stay focused while coding. By starting with a failing test, you concentrate solely on making it pass. This approach encourages thinking in terms of smaller chunks of code, rather than tackling the entire function at once.
- Fewer Bugs
It can be incredibly frustrating to discover bugs after building an entire function. Finding the problem and determining when it occurred becomes challenging. However, by practising TDD and frequently testing small blocks of code, you can reduce the occurrence of bugs. This approach helps identify and resolve issues before the function grows in complexity.
3. Unit Test Process with an Example
Let’s delve into how the TDD process works with a simple example.
- Write a test
To demonstrate the TDD process, let’s consider a simple example where we want to test a function called addNumber(). We will write a test to verify that the function correctly adds two numbers.
function addNumber() {
if (add(4, 5) === 9) {
console.log("TEST PASSED");
} else {
console.log("TEST FAILED");
}
}
In this test, we expect the addNumber() function to call another function named add() with the parameters 4 and 5. We then check if the result is equal to 9. If the condition is true, we log “TEST PASSED”, otherwise, we log “TEST FAILED”.
2. Write a code
We write the code for the add() function to make the test pass.
function add(a, b) {
return a + b;
}
The add() function takes two parameters, a and b, and returns their sum.
3. Run the test and Observe Success
addNumber()
After implementing the add() function, we rerun the test and observe that it passes successfully. The result of add(4, 5) is indeed 9.
4. Refactor (if needed)
If there is a need for refactoring, this step allows us to improve the code structure without changing its behaviour. In this simple example, there might not be a need for significant refactoring.
By following the TDD process, we ensure that our code is developed incrementally and tested thoroughly. This iterative approach helps identify and address issues early on, leading to more reliable and maintainable code.
4. Conclusion
Test Driven Development (TDD) is a valuable practice that promotes code reliability and logical thinking. By writing tests first, we can focus on smaller units of code, leading to fewer bugs and better maintainability. The TDD process, as demonstrated with the example of testing the addNumber() function, allows us to incrementally build and test our code, ensuring that the desired functionality is achieved.
By adopting TDD, developers can enhance the quality of their code, improve productivity, and build robust software that meets the desired requirements. So why not give TDD a try in your next project and experience its benefits firsthand? Happy coding!